Declaring an int allocates 4 bytes or 32 bits of memory
This allows for storing numbers up to or
Why not ?
Integers are exact, so can be safely used for equality comparisons
BUT if you exceed the maximum value, you get integer overflow:
int i = 2147483647;
i = i + 1;
cout << i << endl; // -2147483648
Debugging with gdb
In tomorrow's lab, you will be introduced to the GNU Debugger gdb
gdb is a command-line tool that allows you to:
Run your program line-by-line
Inspect the values of variables
Set breakpoints to pause execution
And much more!
To build with debug info (such as line numbers) use the -g flag:
g++ -g hello.cpp
gdb demo
After building with -g, run gdb on the executable:
gdb ./a.out
You will see a (gdb) prompt
Type run to start the program - this will run the whole thing
Type list to see the source code
To add a breakpoint, type b <line number> (or break <line number>), e.g.:
b 7
Now run again, and the program will pause at line 7
Basic gdb commands
Command
Description
run
Run the program
list
List the source code
b <line number>
Set a breakpoint at the given line number
d <breakpoint number>
Delete the given breakpoint
n
Execute the next line of code
p <variable name>
Print the value of the given variable
c
Continue execution until the next breakpoint
Coming up next
Lab: C++ and gdb
Lecture: Using and defining functions in C++
Textbook Chapter 4 and start of 5
Do a demo with and without const, try to modifiy
## Constants using `#define`
C++ also has a preprocessor directive called `#define`:
```cpp
#define PI 3.14159
#define GST 0.05
#define NUM_PLANETS 8
```
* Preprocessor directives are processed **before** the code is compiled
* `#define <name> <value>` performs **text replacement** of the name with the value
<div data-marpit-fragment>
> `const` or `#define`? Subtle differences, but as usual, **be consistent**
</div>
More demos
const has type correctness and scope
Demo demo demo
Draw the stream concept on the board
show with and without the newline
## 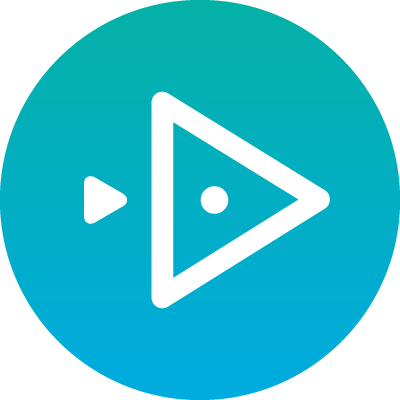 What does the following display?
<div class="columns">
<span class="Alpha">
1.
```
Hello, world!
123
Aa
```
2.
```
Hello, world!
1 2 3
A a
```
3.
```
Hello, world! 1 2 3
A a
```
4.
```
Hello, world!123
Aa
```
</span>
```cpp
int main()
{
int number = 3;
cout << "Hello, world!";
cout << 1 << 2 << number << endl;
cout << 'A' << 'a' << endl;
return 0;
}
```
</div>
Move to example, show how whitespace is not needed for characters, but needed to separate numbers
Draw a diagram of chopping up the input stream and feeding it to chained variables
Show what happens with the sign bit (1 for negative)